Every Android app project must include an AndroidManifest.xml
file in the root of the project source set. The manifest file informs the Android build tools, the Android operating system, and Google Play with important information about your app.
Among other things, the manifest file must specify the following:
- All the app's functions, services, broadcast receivers, and content producers are its components. Each component must specify fundamental attributes like the name of its Java class. Additionally, it can list capabilities like the device settings it can manage and intent filters that specify how the component can be launched.
- The permissions that the app needs in order to access the system or other applications' secure areas. Additionally, it specifies any rights that must be granted for other applications to access material from this app.
- Which devices can download the app from Google Play depends on the hardware and software features the app needs.
Most of the necessary manifest items are added while you develop your app if you're using Android Studio to construct the manifest file (especially when using code templates).
Android Manifest File: Examples, relevance and location
Every Android project has an AndroidManifest.xml
file, which is kept in the root directory of the project hierarchy. The manifest file is a crucial component of our program since it specifies the metadata, needs, and application structure. WorkspaceName>/temp/AppName>/build/luaandroid/dist
is where you may find the file. The manifest file gives the Android operating system and Google Play Store crucial information about your app. The permissions that an app needs to have in order to access data from other applications are declared within the Android manifest file.
What is an Android application manifest file?
Information about an Android application is contained in the Android App Manifest. At the base of the source set, there is a single AndroidManifest.xml
file for each application. Android App Manifests are used by the Android operating system and digital distribution platforms (like Google Play) to locate details about an application, including its name, entry point, support for hardware features, Android version, and permissions. See the Android Developer documentation on Android App Manifests for further details about the Android App Manifest file and a list of the parameters that it configures.
The program metadata, which includes the icon, version number, themes, etc., is included in the manifest file. Additional top-level nodes can also provide any necessary permissions, unit tests, and hardware, screen, or platform requirements. A root manifest tag with the project's package as its package attribute makes up the manifest. Additionally, an xmls:android
attribute that provides a number of system attributes utilized inside the file should be included. The versionCode
property is internally used to specify the current application version as a number that automatically increases with each repetition of the version that has to be updated. A public version that will be shown to users is also specified using the versionName
parameter.
Here are some of the most important points:
- It specifies the application's Java package name. The package name acts as the application's special identification.
- It outlines the elements that make up the application, including its functions, services, broadcast receivers, and content suppliers. Each component's implementation classes are shown, along with its capabilities (for example, which Intent messages they can handle). The Android system may learn more about the components and the circumstances in which they might be launched thanks to these declarations. More of these will be further discussed in this article.
Structure of the manifest file
The structure of the file usually takes the form of a code-like instruction and looks usually like this:
<manifest>
<application>
<activity
android:name="com.example.applicationname.MainActivity">
</activity>
</application>
</manifest>
The following data is contained in the manifest file:
- The name of the application's package, often known as the namespace of the code. As the project is being built, this information is utilized to locate the code.
- The component that consists of all the actions, services, recipients, and content providers is another.
- The permissions that the application needs in order to access the system's and other apps' protected areas.
- Which devices can download the app from Google Play depends on the functionality that the app needs. Both hardware and software features are included in these features.
- Additionally, it contains information about the program metadata, such as the icon, version, themes, etc.
Furthermore, here are some other things it does:
- It selects the processes that will host the various application components.
- It specifies which rights are necessary for the application to communicate with other programs and access restricted areas of the API.
- It includes a list of the instrumentation classes, which offer profiling and other data while the program is operating. These declarations are deleted from the manifest before the application is released and are only present there while the application is being built and tested.
- It specifies the Android API level that the application must have at a minimum.
- It also specifies which libraries that the program must be linked against
Typically, the android manifest.xml file looks like this:
<?xml version="1.0" encoding="utf-8"?>
<manifest
xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.myfirstapplication">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity
android:name=".MainActivity">
<intent-filter>
<action
android:name="android.intent.action.MAIN" />
<category
android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<meta-data
android:name="preloaded_fonts"
android:resource="@array/preloaded_fonts" />
</application>
</manifest>
How to edit Android manifest file
To make modifications to your file, follow these steps;
Update the AndroidManifest.xml
file to include a Detail Activity class. When you test the program, this declaration allows the application to launch a customer detail screen where you may make adjustments.
- Double-click the
AndroidManifest.xml
file in Package Explorer. - The
AndroidManifest.xml
tab should be selected. - Fill up the
AndroidManifest.xml
file with these settings. To copy and paste the full<activity>
element, utilize theAndroidManifest.xml
file contained in the ZIP archive:
<activity
android:name=".DetailActivity"
android:label="@string/app_name">
<intent-filter>
<action
android:name="android.intent.action.MAIN" />
<category
android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
1. choose File > Save
Element tags of manifest file
A manifest file contains the nodes that specify the test classes, requirements, security settings, and application components. Among the most common manifest sub-node tags are:
<manifest>
It is this element's root component. It is made up of a package attribute package that specifies the name of the activity's package.
<application>
The namespace declaration is contained in the subclass of the manifest file. It has several characteristics. These properties identify the components of the application, and they comprise:
- icon
- allowBackup
- label
- theme
<activity>
Activity is a component of each application. The statement of the activity that is required in the manifest file is included there. Additionally, it contains characteristics like a name, label, theme, etc.
<intent-filter>
It specifies the kind of intent that the Android components can respond to and is a component of the activity.
<action>
This component gives the intent filter an action. At least one active element is required for each intent filter.
<category>
The category name is added by this element to an intent filter.
<service>
The operations offered by libraries or APIs are included in this element.
How to define the app components?
The fundamental building blocks of an Android app are called app components. In the manifest file, you must specify a corresponding XML element for each app component you build in your project.
Each element serves as a point of entry for the system or a user to access your program. Some elements rely on other elements.
Four main categories of app components exist:
<activity>
for each subclass of Activity.<service>
for each subclass of Service.<receiver>
for each subclass of BroadcastReceiver.<provider>
for each subclass of ContentProvider.
Each type has a unique lifetime that outlines how the component is formed and destroyed, in addition to serving a specific purpose. The four categories of app components are described in the sections that follow.
How to set manifest file permissions in Android?
To access other Android applications or the Internet, an app must first get certain permissions. The manifest file, manifest.xml, is automatically created when our program is being built. We have specified the permissions in this manifest file. If no permission is given by us, a few permissions are automatically applied.
The users have the option of accepting or rejecting the permissions listed in the manifest file. The protected features can be used by the program after the user gives it permission to do so. The user must provide permission for the app to access the protected features; otherwise, it will not.
By default, the following permissions are included and are turned to TRUE:
INTERNET
ACCESS_NETWORK_STATE
READ_PHONE_STATE
How to ensure device compatibility?
You may specify in the manifest file what features—hardware or software—your program needs, as well as the devices with which it is compatible. The Google Play Store forbids the installation of your program on devices with insufficient system capabilities or features.
The various manifest tags specify the devices with which your program is compatible. Here are just a few of the most popular tags:
<uses-feature>
<uses-sdk>
<uses-configuration>
<supports-screens>
<permission-groups>
File conventions
This section discusses the standards and regulations that typically apply to all elements and attributes in the manifest file.
ElementsIt is just necessary to provide the manifest and application parts. Each one can only happen once. The majority of the other components can happen zero times or more. But for the manifest file to be useful, at least some of them must be there. Instead of being stored as character data inside an element, all of the values are set using attributes.
In general, elements at the same level are not arranged. The activity>, provider>, and service>components, for instance, can be arranged in any sequence. This rule has two important exceptions:
- An element with the name "activity-alias" must come after the activity it is an alias for.
- The final element inside the manifest element must be the application> element.
Attributes
Technically, every characteristic is up for grabs. However, in order for an element to serve its intended purpose, a number of characteristics must be provided. The reference documentation lists the default values for properties that are actually optional.
All attribute names start with an android: prefix, with the exception of a few attributes of the root <manifest>
element. Android:alwaysRetainTaskState
, as an example. The documentation often omits the prefix when referring to characteristics by name because it is ubiquitous.
Multiple values
Instead of listing several values inside a single element when more than one value may be given, the element is nearly always repeated.
Resource values
Users can see the values of some properties, such as the title of an activity or the icon for your app. The settings should be set from a resource or theme rather than being hard-coded into the manifest file since they may vary depending on the user's language or other device parameters (such as to give a varied icon size dependent on the device's pixel density). Based on alternative resources you give for various device setups, the actual value may then shift.
String values
When an attribute value is a string, you must escape characters like n for a newline or uxxxx for a Unicode letter using double backslashes().
File features
The next sections go through how the manifest file reflects some of your app's most crucial features.
Intent filters
Intentions turn on services, broadcast receivers, and app actions. An intent is a message specified by an intent object that specifies an action to be taken. It includes information about the data to be acted upon, the type of component that should carry out the action, and other directives. Based on intent filter declarations in each app's manifest file, the system determines which app component may handle an intent when an app issues one to the system. The matching component is launched by the system, and it is provided with the Intent object. The user can choose which app to use if more than one app can handle the purpose.
Icons and labels
Several components have icon and label properties, allowing users to see a tiny icon and a written label. Others feature a description element for more detailed explanations that can be shown on-screen. A permission symbol, its name, and an explanation of what it implies can all be displayed to the user when they are asked whether to give permission to an application that has requested it, for instance, since the element contains all three of these properties.
Any time an icon or label is set in a contained element, those changes are applied automatically to all of the container's child components. The icon and label specified in the application>
element serve as the application's default icons and labels for all other components. The icon and label chosen for a component, such as an "activity" element, serve as its default settings for all "intent-filter" components. When activity and its intent filter do not set a label, but an application element does, the application label is assumed to be the label for both the activity and the intent filter.
Permissions
A permission is a restriction that restricts access to a specific section of the device's code or to certain data. The restriction is put in place to safeguard crucial data and code that may be used improperly to taint or harm the user experience. Each permit has its own distinct label to identify it. Frequently, the label identifies the prohibited conduct.
As of Android 6.0 (API level 23), the user has the option to accept or deny certain app permissions at runtime. However, you must specify all permission requests using a <uses-permission>
part in the manifest regardless of whatever Android version your app supports. The protected functionalities can be used by the app if permission is granted to do so.
Otherwise, attempts to access such functions are unsuccessful. Additionally, your program can use permissions to safeguard its own parts. Any permission specified by Android, as described in android, may be used. A permission that has been declared in another app or manifest.permission
. Additionally, your app can set its own permissions. The permission>
element announces a new permission.
Libraries
Each program is linked to the standard Android library, which contains the fundamental packages for creating apps (with common classes such as Activity, Service, Intent, View, Button, Application, ContentProvider, and so on).
Some packages, however, are housed in separate libraries. Your application must expressly request to be linked against these packages if it requires any code from any of these packages. Each library's name must appear in its own uses-library>
element in the manifest. (The package documentation contains the library's name.)
Summary
You gained knowledge about the Android Manifest file and its purpose. You saw the format and contents of a manifest file. Later, you discovered components in the Manifest file and learned what each one did. The process for granting permission in your app using the Manifest file was then shown to you in detail.
Build unique products, boost device performance
See why emteria is the chosen Android™ customization & management platform for OEM solution builders — and what it can do for your team and customers.
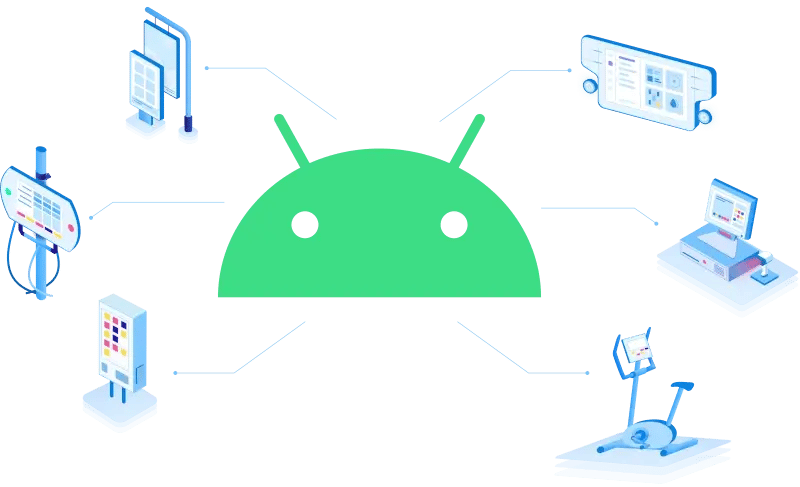